Context
I have a lot of pictures on my computer. One of my hobbies is following japanese artists on twitter who draw art of my favorite characters and sorting it into my personal archive. About a year ago or so twitter started changing all their image files from JPG to JFIF and I wrote myself a quick and dirty scala program to walk a file tree and change the extension of each of those files and then updated my right click context menu so I could run it anywhere to fix things up.
Fast forward a number of mandatory, annoyingly scheduled, and unneccesary windows updates later and my registry key stopped working for some reason and I let things slip for a while on renaming files and making sure that when I was sharing a picture from an artist the file was a .jpg so it would actually render in the preview for discord.
Ren is slow as shit.
I was doing this and dumping a bunch of pictures to a friend of mine who I like to gush to about my love of one particular character, and decided it was time to do a rename. Out of curiousity, I did a quick search on the internet and found myself here. Which of course led me to the very useful command to rename all the file extensions in a directory like I wanted:
ren *.jfif *.jpg
Excited I wouldn't have to dig into the world of windows context menu setup and the registry, I happily typed the command into cmd.exe and then went to grab a drink from the fridge.
I came back about a minute later and was confused to see this:
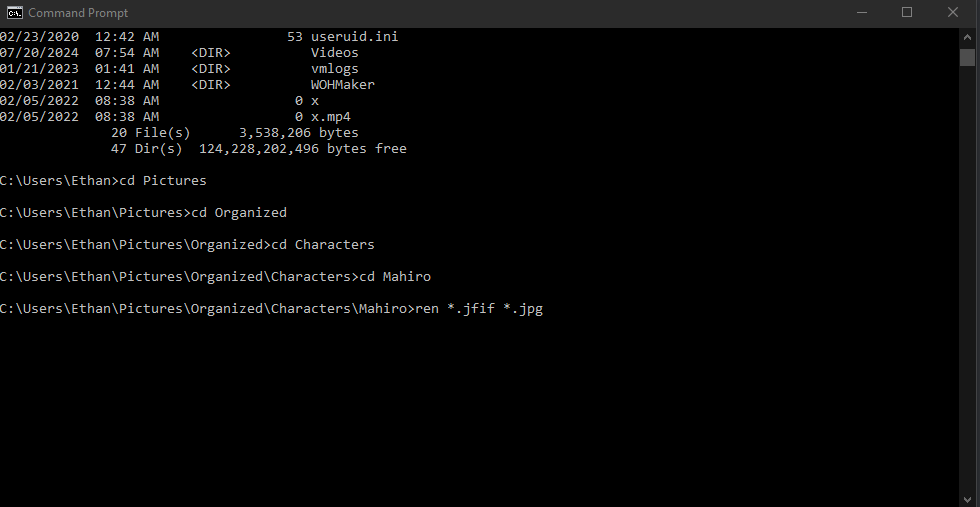
Somewhat confused, I opened up the folder in question and saw that according to the properties files of the directory
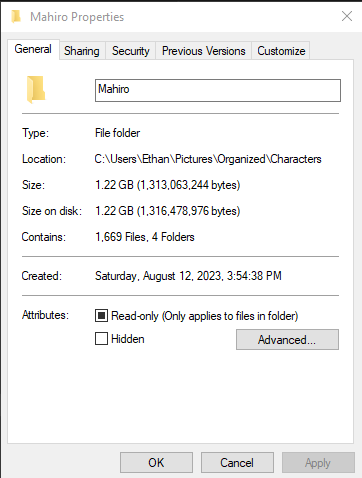
I opened the folder and observed that, yes, I was pretty sure some of the jfif files had been renamed, but scrolling the folder showed plenty of files which still needed help. I scrolled up until I found the border between the two extensions and observed that nothing was changing. I had expected to perhaps see a slow one-by-one rename of the files I could see in explorer. Nope. Just a stalled out command line doing nothing but spinning.
I opened the task manager to see if it was stuck somewhere and saw this
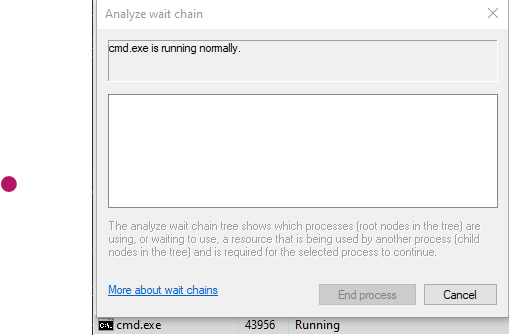
So... cmd.exe isn't waiting on any resources or locks or anything like that. It was also only taking up 0% of the cpu and 2.4MB of memory.
I can fix this
My goal isn't to fix windows. My goal is to rename all the shitty files from twitter to an extension that normal human beings can enjoy. I've coded it once, I can code it again. So, I gave myself a quick cheeky task:
"Can I write a script in Java to do this file rename faster than it takes for cmd.exe to finish?"
I am happy to report that the answer is yes. I popped open Intelliji and created a new project.
Java has a lovely visitor pattern style approach to walking file trees, and it makes for some pretty simple coding. I could refactor this to be nicer, and maybe I will, but my initial code was just this:
import java.io.IOException; import java.nio.file.Files; import java.nio.file.Paths; import java.nio.file.Path; import java.nio.file.SimpleFileVisitor; import java.nio.file.FileVisitResult; import java.nio.file.attribute.BasicFileAttributes; import java.nio.file.StandardCopyOption; public class Main { public static void fixFile(Path path) throws IOException { if (path.getFileName().toString().endsWith(".jfif")) { String[] parts = path.toAbsolutePath().toString().split(".jfif"); Path newPath = Paths.get(parts[0] + ".jpg"); Files.move(path, newPath, StandardCopyOption.REPLACE_EXISTING); } } public static void main(String[] args) throws IOException { for (int i = 0; i < args.length; i++) { String filename = args[i]; Path originalFile = Paths.get(filename); if (originalFile.toFile().exists()) { if (originalFile.toFile().isDirectory()) { Files.walkFileTree(originalFile, new SimpleFileVisitor<Path>() { @Override public FileVisitResult visitFile(Path file, BasicFileAttributes attrs) throws IOException { if (attrs.isRegularFile()) { fixFile(file); }; return FileVisitResult.CONTINUE; } }); } else { fixFile(originalFile); } } else { System.out.printf("File does not exist: %s%n", filename); } } } }
Rather than spend the extra time to get it wired up in a context menu and dive into the windows keys, I elected to just run the rename from Intelliji:
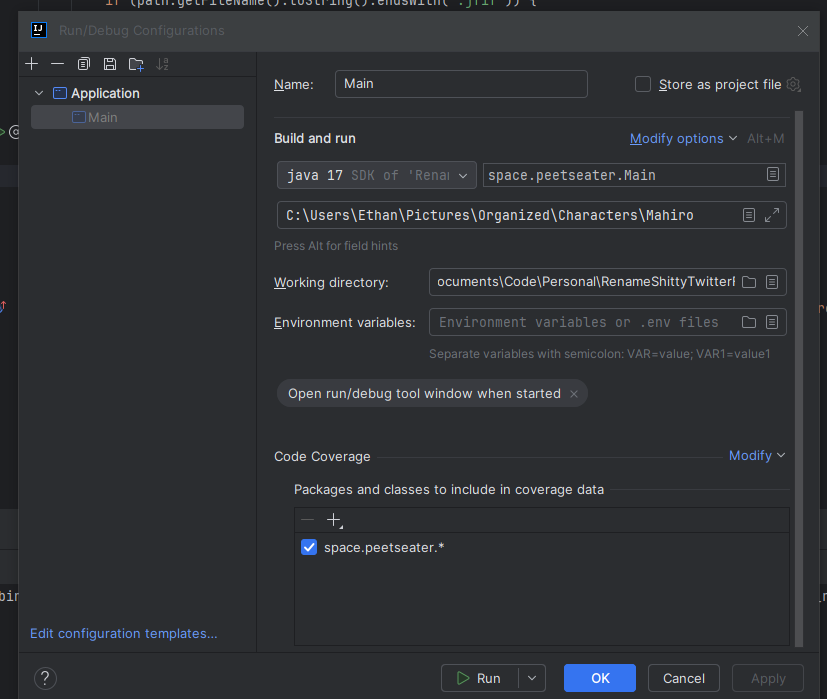
A few microseconds later Process finished with exit code 0
showed up in the run window of Intelliji and
I looked over at the ren command in the cmd.exe terminal:
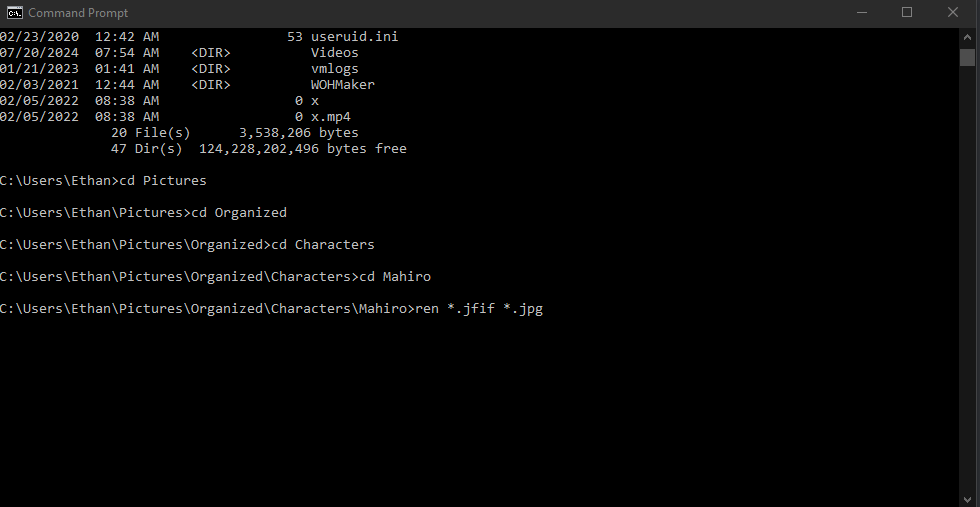
Amazingly, it was still running. Now, I know there's got to be something wrong with my system. Maybe the way windows is adding files to its search index? I don't think that's it because I very explicitly told windows NOT to index my pictures folder because I can handle organizing and finding the files myself without its help. So, I went ahead and threw out a search to the internet and was unsurprised to find that other people have problems with the renaming in windows:
- Rename is slow during right click on windows 10
- Someone on reddit complaining
- Windows 10 forums about not just renaming, but deleting and creating files
- Some people complaining about this on windows 11 and talking about hardware corruption
I could keep showing you more search results, but you can find them yourself. So. Feeling not alone, I decided to do one more race.
Could I finish writing up a blog post and sharing the utility code I just wrote with the world via github
before the ren
command finished?
A picture is worth 1000 words
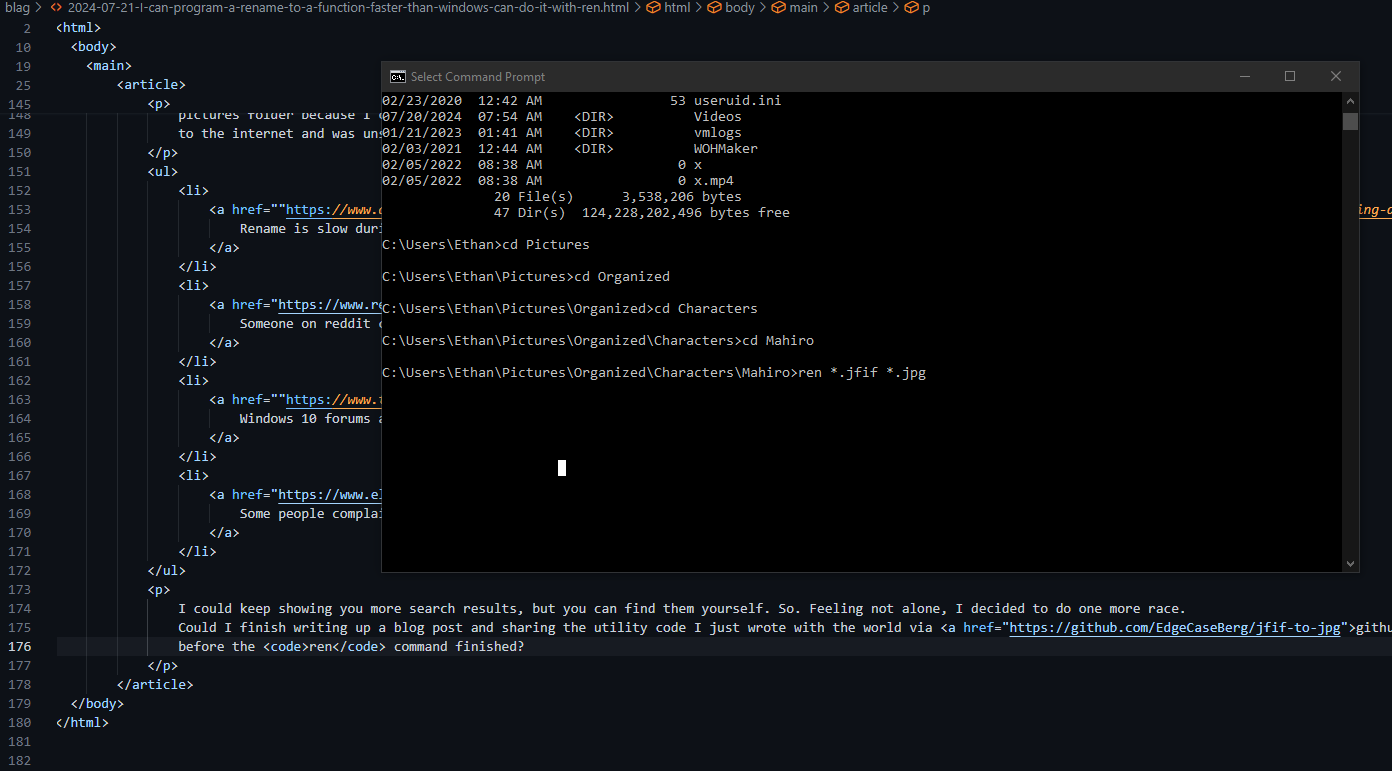